How to Setup a Maven Project in Intellij
What is Maven?
Maven is a tool that helps developers manage their projects, mainly for Java applications. It’s primarily a build and project management tool for Java, but it can also work with other JVM-based languages like Kotlin and Scala. Maven makes it easy to download the libraries your project needs, organize your project files, and automate the steps to compile your code and create an executable file. This means you can focus more on writing your code instead of worrying about setting up your project.
Regardless of the framework you choose, Maven can simplify the management of your application’s build process. By using Maven, developers don’t have to worry about finding and installing each library manually, which saves time and reduces mistakes. Overall, Maven helps developers focus more on writing their code instead of getting caught up in the details of setting up their projects.
Example: Adding a Dog Object to the Spring Context
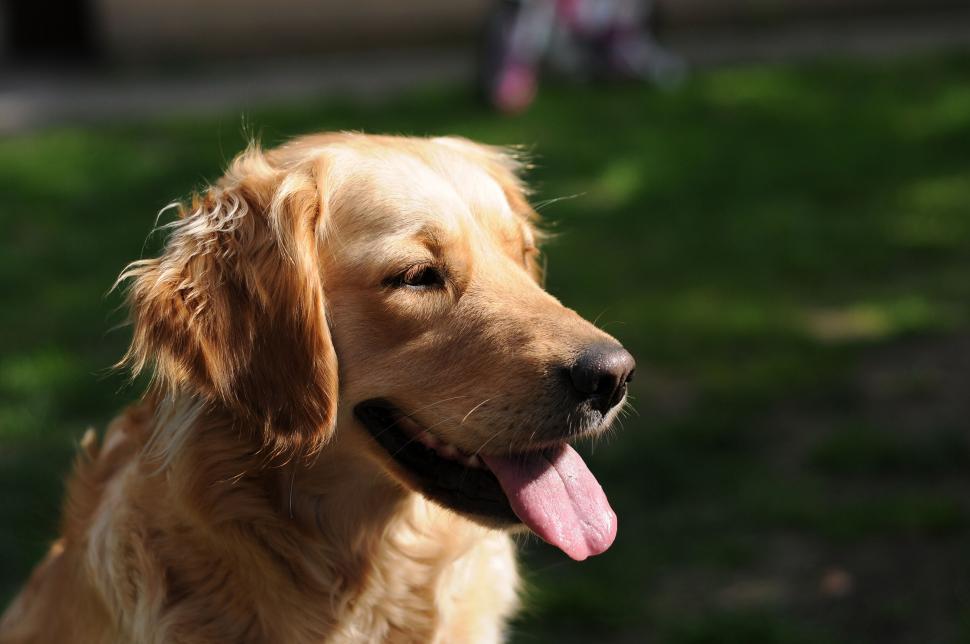
Let’s go over a simple example of adding a Dog object to the Spring context. This will demonstrate how Maven simplifies dependency management and application setup. Here is the code we’ll be executing:
@SpringBootApplication
public class MainApplication {
public static void main(String[] args) {
ApplicationContext context = SpringApplication.run(MainApplication.class, args);
Dog dog = context.getBean(Dog.class);
System.out.println(dog);
}
@Bean
public Dog dog() {
return new Dog("Charlie", 2);
}
}
public class Dog {
private String name;
private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Dog{name='" + name + "', age=" + age + "}";
}
}
In this code, we define the Main class as the entry point for our Spring Boot application. We create a Dog bean with the name “Charlie” and age 2, which is then retrieved from the application context and printed to the console.
Without Maven
If you choose not to use Maven, you would need to manually manage all the dependencies and libraries required for your project. This includes downloading the necessary JAR files for Spring Boot and any other libraries your application might use. For instance, you will most likely need the following JARs:
The spring-boot-3.3.4.jar
is the main Spring Boot JAR. The spring-core-6.1.13.jar
contains core spring functionalities and the spring-beans-6.1.13.jar
is used for managing beans in the Spring context. These JARs are essential for any Spring Boot application, but there are many others that you may need, depending on your project’s requirements.
For example, if you wanted to install the spring-boot-starter-3.3.4.jar
, you would go to the Maven repository here and manually download the JAR file.
Without Maven, you’d need to download all these dependencies individually like this, ensuring that you match the correct versions to avoid compatibility issues. You would then manually add JARs to your project’s build path in your IDE. This tells your IDE or the JVM where to find the dependencies needed for compiling and running your project. This process can be tedious and error-prone, especially as your project grows in complexity.
With Maven
When using Maven with Spring Boot, the process of downloading JAR files is fully automated. By defining your project’s dependencies in a pom.xml
file, Maven automatically downloads the required libraries. For instance, when you include the spring-boot-starter dependency, Maven not only downloads the Spring Boot starter JAR but also its transitive dependencies (like spring-core and spring-beans). This ensures that the correct versions are used, reducing the risk of version conflicts and simplifying your project setup. However, Maven is useful for much more than just dependency management. It can automate other tasks in the build process including compiling the app, packaging the app in an executable archive like a JAR or WAR file, running tests, and more.
Let’s walk through this code using Spring Boot from Spring Initializr and Intellij.
Before we start setting up the project, let’s go over a few important terms in Maven.
Group:
- This is a name that helps identify your project or organization. It usually looks like a website address written backward (for example,
com.example
). It helps keep your projects organized and easy to find.
Artifact Name:
- This is the name of your project. It’s like naming what you’re building (for example,
my-app
). This name helps identify your project when it’s created or shared.
Package Name:
- This organizes your Java files. It usually matches the group name (like
com.example.main
). It helps keep your code organized and avoids confusion with other projects.
We’ll add the web dependency now. While we don’t specifically need the web features, it includes the Spring Boot starter, which is what we actually require. After adding the web dependency, click on “Generate” to download your project files.
Once you’ve downloaded the project, extract the zip file. Then, open IntelliJ and select File > Open. Navigate to the extracted folder and open it to load your project in IntelliJ.
In a Maven project created through Spring Initializr and opened in IntelliJ, the folder structure is designed for clarity and organization. Here’s how it breaks down:
src/main/java
: This is where your main application code goes. You’ll put your Java classes in this folder, following your package name structure (likecom.example.main
). It helps keep your code organized.src/main/resources
: This folder contains non-Java files your application needs, like configuration files (e.g., application.properties orapplication.yml
), templates, and static assets. It holds all the important files that aren’t Java code.src/test/java
: This directory contains your test classes and mirrors the structure of src/main/java. For each class insrc/main/java
, you can create a corresponding test class here. This alignment makes it easy to manage your tests alongside your main code.pom.xml
: This is the central configuration file for your project. The pom.xml lists your project’s dependencies, plugins, and settings. Maven uses it to manage the build process and dependencies.
This folder structure is specific to Maven projects, and Maven manages your dependencies by installing them from the Maven Repository. These dependencies get installed into the External Libraries folder within your project in IntelliJ. This folder provides easy access to all the libraries and their transitive dependencies that your project relies on.
Running the program is as simple as clicking the green play button in IntelliJ. Once you do that, you’ll see your application in action, showcasing the features you’ve implemented.
To wrap things up, setting up a Maven project with Spring Boot in IntelliJ streamlines dependency management and keeps your code organized. With this solid foundation established, you can now focus on building and enhancing your applications.